프린트 보조 스트림
PrintStream과 PrintWriter는 프린터와 유사하게 출력하는 print(),println() 메소드를 가지고 있는 보조 스트림이다.
PrintStream은 바이트 기반 출력 스트림과 연결되고, PrintWriter는 문자 기반 출력 스트림과 연결 된다.
System.out.println();이 바로 PrintStream 타입이다.
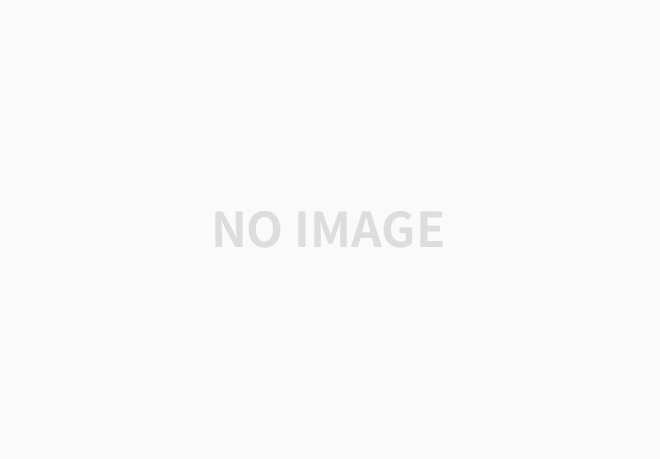
package sec03.exam01;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintStream;
public class PrintStreamEx {
public static void main(String[] args) throws FileNotFoundException {
// 바이트 기반 출력 스트림을 생성하고 printstream 보조 스트림 연결
File file = new File("C:/Temp/printstream123.txt");
FileOutputStream fos = new FileOutputStream(file);
PrintStream ps = new PrintStream(fos);
// 파일, 폴더 존재 여부 확인
if(file.exists()) {
System.out.println("있다.");
}else {
System.out.println("없다.");
}
// printstream123.txt 해당 파일에 데이터 입력
ps.println("[프린터 보조 스트림]");
ps.print("마치");
ps.println("프린터가 출력하는 것처럼");
ps.println("데이터를 출력합니다.");
ps.flush();
ps.close();
}
}
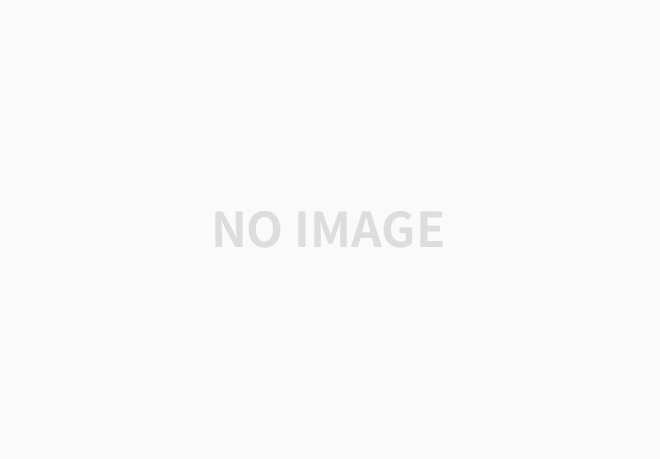
객체 입출력 보조 스트림
ObjectOutputStream과 ObjectInputStream 보조 스트림을 연결하면 메모르에 생성된 객체를 파일 또는 네트워크로
출력이 가능 하다.
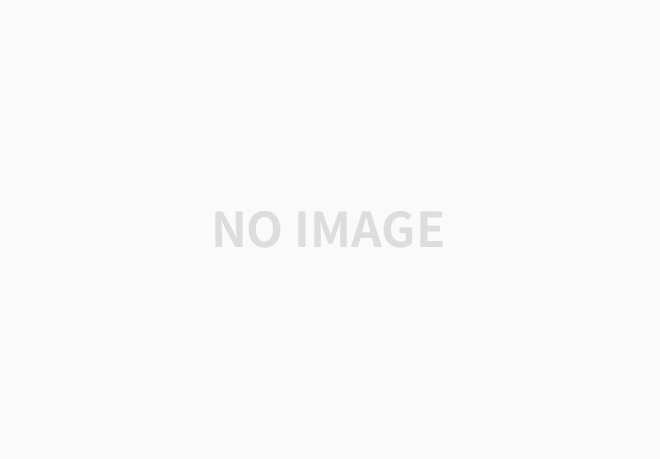
ObjectOutputStream은 객체를 직렬화하는 역할을 하고, ObjectInputStream은 객체로 역직렬화하는 역할을 한다.
직렬화란 객체를 바이트 배열로 만드는 것이고, 역직렬화란 바이트 배열을 다시 객체로 복원하는 것을 말한다.
ObjectInputStream ois = new ObjectInputStream(바이트 기반 입력 스트림);
ObjectOutputStream oos = new ObjectOutputStream(바이트 기반 입력 스트림);
두개는 다른 보조 스트림과 마찬가지로 연결할 바이트기반 입출력 스트림을 생성자의 매개값으로 받는다.
oos.writeObject(객체);
ObjectOutputStream의 writeObject( ) 메소드는 객체를 직렬화해서 출력 스트림으로 보낸다.
객체타입 변수 = (객체타입) ois.readObject();
반대로 ObjectInputStream의 readObject( ) 메소드는 입력 스트림에서 읽은 바이트를 역직렬화해서
객체로 다시 복원해서 리턴한다. 리턴 타입은 Object 타입이기 때문에 원래 타입으로 위에 처럼 강제 변환
해야 한다.
자바는 모든 객체를 직렬화하지 않는다. java.io.Serializable 인터페이스를 구현한 객체만 직렬화한다.
Serializable 인터페이스는 메소드 선언이 없는 인터페이스이다. 객체를 파일로 저장하거나, 네트워크로 전송할
목적이라면 개발자는 클래스를 선언할 때 implements Serializable을 추가해야 한다.
이것은 개발자가 JVM에게 직렬화해도 좋다고 승인하는 역할을 한다고 보면 된다.
밑에 예제를 보고 확인
Serializable 인터페이스 구현
package sec03.exam03;
import java.io.Serializable;
import java.util.Date;
// Serializable 인터페이스 구현
public class Board implements Serializable {
private int bno;
private String title;
private String content;
private String writer;
private Date date;
public Board(int bno, String title, String content, String writer, Date date) {
super();
this.bno = bno;
this.title = title;
this.content = content;
this.writer = writer;
this.date = date;
}
public int getBno() {
return bno;
}
public void setBno(int bno) {
this.bno = bno;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public String getWriter() {
return writer;
}
public void setWriter(String writer) {
this.writer = writer;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
}
실행
package sec03.exam03;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class ObjectStreamEx {
public static void main(String[] args) throws IOException, ClassNotFoundException {
writeList(); // List를 파일에 저장
List<Board> list = readList(); // 파일에 저장된 List 읽기
// List 내용을 모니터에 출력
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
for(Board board : list) {
System.out.println(
board.getBno() + "\t" + board.getTitle() + "\t" +
board.getContent() + "\t" + board.getWriter() + "\t" +
sdf.format(board.getDate())
);
}
}
public static void writeList() throws IOException {
List<Board> list = new ArrayList<Board>();
// list에 Board 객체 저장
list.add(new Board(1, "제목1", "내용1", "글쓴이1", new Date()));
list.add(new Board(1, "제목2", "내용2", "글쓴이2", new Date()));
list.add(new Board(1, "제목3", "내용3", "글쓴이3", new Date()));
// 객체 출력 스트림을 이용해서 list 출력
FileOutputStream fos = new FileOutputStream("c:/Temp/board.db");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(list);
oos.flush();
oos.close();
}
// 객체 입력 스트림을 이용해서 list 읽기
public static List<Board> readList() throws IOException, ClassNotFoundException {
FileInputStream fis = new FileInputStream("c:/Temp/board.db");
ObjectInputStream ois = new ObjectInputStream(fis);
List<Board> list = (List<Board>) ois.readObject();
return list;
}
}
<결과>
1 제목1 내용1 글쓴이1 2023-06-28
1 제목2 내용2 글쓴이2 2023-06-28
1 제목3 내용3 글쓴이3 2023-06-28
File 클래스
java.io 패키지에서 제공하는 File 클래스는 파일 및 폴더 정보를 제공해주는 역할을 한다.
package sec03.exam02;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class FileEx {
public static void main(String[] args) throws IOException {
File dir = new File("C:/Temp/images"); // 디렉터리 생성
File file1 = new File("C:/Temp/file1.txt");// File 객체 생성
File file2 = new File("C:/Temp/file2.txt");
File file3 = new File("C:/Temp/file3.txt");
// 파일 또는 폴더가 존재하지 않으면 생성
if(dir.exists() == false) {dir.mkdirs();}
if(file1.exists() == false) {file1.createNewFile();}
if(file2.exists() == false) {file2.createNewFile();}
if(file3.exists() == false) {file3.createNewFile();}
// C:/Temp 폴더의 내용 목록을 File 배열로 얻음
File temp = new File("C:/Temp");
File[] contents = temp.listFiles();
System.out.println("시간\t\t\t형태\t\t크기\t이름");
System.out.println("-----------------------------------");
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd a HH:mm");
for(File file : contents) {
// 파일 또는 폴더 정보를 출력
System.out.println(sdf.format(new Date(file.lastModified())));
if(file.isDirectory()) {
System.out.println("\t<DIR>\t\t\t" + file.getName());
}else {
System.out.println("\t\t\t" + file.length() + "\t" + file.getName());
}
System.out.println();
}
}
}
<결과>
시간 형태 크기 이름
-----------------------------------
2023-06-28 오후 16:33
382 board.db
2023-06-28 오전 10:13
6 file1.txt
2023-06-28 오전 09:33
0 file2.txt
2023-06-28 오전 09:33
0 file3.txt
2023-06-28 오전 09:33
<DIR> images
2023-06-28 오후 16:17
104 printstream123.txt

File 클래스를 이용한 파일 및 폴더 정보 출력
package sec03.exam02;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.stream.Stream;
public class FromFileEx {
public static void main(String[] args) throws IOException {
Path path = Paths.get("C:\\Temp\\file1.txt");// 객체 생성
// Files.lines() 메서드를 사용하여 path에 지정된 파일을 열고, 각 줄을 Stream으로 읽음
Stream<String> stream = Files.lines(path, Charset.defaultCharset());
// stream 객체를 사용하여 각 줄을 반복하며, 각 줄을 콘솔에 출력
stream.forEach(s->System.out.println(s));
// 파일을 객체로 변환
File file = path.toFile();
// FileReader 객체를 사용하여 file을 읽음
FileReader fileReader = new FileReader(file);
// fileReader를 사용하여 파일을 읽음
BufferedReader br = new BufferedReader(fileReader);
// BufferedReader 객체 br을 사용하여 각 줄을 Stream으로 읽어옴
stream = br.lines();
// 각 줄을 반복하며, 각 줄을 콘솔에 출력
stream.forEach(s->System.out.println(s));
Path path2 = Paths.get("c:/Temp");
// Files.list() 메서드를 사용하여 path2에 지정된 디렉토리의 파일 목록을 가져옴
Stream<Path> stream2 = Files.list(path2);
stream2.forEach(p->{
System.out.println(p.getFileName());
});
}
}
<결과>
파일
파일
file1.txt
file2.txt
file3.txt
images
printstream123.txt
실행 결과에 "파일"이 두 번 나오는 이유는 "파일" 이외에도 "images"라는 이름의 디렉토리도 있기 때문입니다.
"images"는 디렉토리이지만, "파일" 출력 후에 "images" 디렉토리의 이름이 출력되므로, "파일"과 "images"가 연이어 나타나는 것
Int Stream, 배열, 일반for문, 향상된 for문,
package sec03.exam02;
import java.util.Arrays;
import java.util.stream.IntStream;
import java.util.stream.Stream;
public class StreamEx {
static int sum = 0;
public static void main(String[] args) {
// 1~10 까지 출력
IntStream st1 = IntStream.rangeClosed(1, 10);
st1.forEach(w->System.out.print(w + ","));// 1,2,3,4,5,6,7,8,9,10
System.out.println();
// 1~10 합계
IntStream st2 = IntStream.rangeClosed(1, 10);
st2.forEach(w->{
sum += w;
});
System.out.println(sum); // 55
// IntStream
int[] intArray = {1,2,3,4,5};
IntStream intStream = Arrays.stream(intArray);
intStream.forEach(a->System.out.print(a + ","));// 1,2,3,4,5
System.out.println();
// 3개 다 결과는 동일
String[] strArray = {"김", "이", "박"};
// Stream
Stream<String> stream = Arrays.stream(strArray);
stream.forEach(s->{
System.out.println(s);
});
System.out.println("-----------------------------");
// 일반 for문
for(int i=0; i < strArray.length; i++) {
System.out.println(strArray[i]);
}
System.out.println("-----------------------------");
// 향상된 for문
for(String str : strArray) {
System.out.println(str);
}
}
}
<결과>
1,2,3,4,5,6,7,8,9,10,
55
1,2,3,4,5,
김
이
박
-----------------------------
김
이
박
-----------------------------
김이박
'프로젝트 기반 자바(JAVA) 응용 SW개발자 취업과정' 카테고리의 다른 글
2023-06-30 31일차 (0) | 2023.06.30 |
---|---|
2023-06-29 30일차 (0) | 2023.06.29 |
2023-06-26 27일차 (0) | 2023.06.26 |
2023-06-22 25일차 (0) | 2023.06.22 |
2023-06-21 24일차 (0) | 2023.06.21 |